# 六、文章搜索
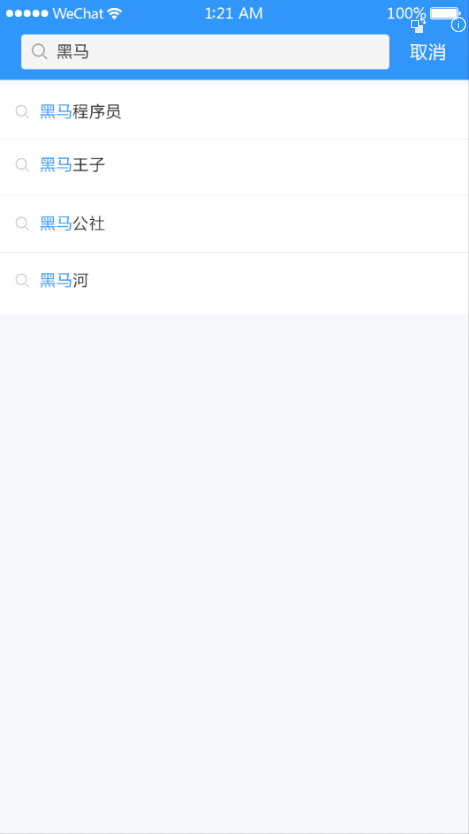
# 1、业务功能分析
# 2、创建组件并配置路由
1、创建 src/views/search/index.vue
<template>
<div class="search-container">搜索页面</div>
</template>
<script>
export default {
name: "SearchPage",
components: {},
props: {},
data() {
return {};
},
computed: {},
watch: {},
created() {},
methods: {}
};
</script>
<style scoped></style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2、然后把搜索页面的路由配置到根组件路由(一级路由)
{
path: '/search',
component: ()=>import('@/views/search')
}
2
3
4
3、首页 搜索按钮,点击跳转
<!-- 导航栏 -->
<van-nav-bar class="page-nav-bar" fixed>
<van-button
class="search-btn"
slot="title"
type="info"
size="small"
round
icon="search"
to="/search"
>搜索</van-button>
</van-nav-bar>
<!-- /导航栏 -->
2
3
4
5
6
7
8
9
10
11
12
13
最后访问 /search
测试。
# 3、页面布局
# 3.1、搜索栏布局
<template>
<div class="search-container">
<!-- 搜索栏 -->
<!--
Tips: 在 van-search 外层增加 form 标签,且 action 不为空,即可在 iOS 输入法中显示搜索按钮
-->
<form class="search-form" action="/">
<van-search
v-model="searchText"
show-action
placeholder="请输入搜索关键词"
background="#3296fa"
@search="onSearch"
@cancel="onCancel"
/>
</form>
<!-- /搜索栏 -->
<!-- 搜索结果 -->
<!-- /搜索结果 -->
<!-- 联想建议 -->
<!-- /联想建议 -->
<!-- 搜索历史记录 -->
<!-- /搜索历史记录 -->
</div>
</template>
<script>
export default {
name: "SearchPage",
components: {},
props: {},
data () {
return {
searchText: '' // 绑定输入框变量
}
},
computed: {},
watch: {},
created() {},
methods: {
onSearch (val) {
console.log(val) // 输入的值
},
onCancel () {
this.$router.back()
}
}
};
</script>
<style scoped lang="less">
.search-container {
padding-top: 108px;
.van-search__action {
color: #fff;
}
.search-form {
position: fixed;
top: 0;
left: 0;
right: 0;
z-index: 1;
}
}
</style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
# 3.2、创建组件
1、创建 src/views/search/components/search-history.vue
<template>
<div class="search-history">
<van-cell title="搜索历史">
<span>全部删除</span>
<span>完成</span>
<van-icon name="delete" />
</van-cell>
<van-cell title="hello">
<van-icon name="close" />
</van-cell>
<van-cell title="hello">
<van-icon name="close" />
</van-cell>
<van-cell title="hello">
<van-icon name="close" />
</van-cell>
<van-cell title="hello">
<van-icon name="close" />
</van-cell>
</div>
</template>
<script>
export default {
name: 'SearchHistory',
components: {},
props: {},
data () {
return {}
},
computed: {},
watch: {},
created () {},
mounted () {},
methods: {}
}
</script>
<style scoped lang="less"></style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
2、创建 src/views/search/components/search-suggestion.vue
<template>
<div class="search-suggestion">
<van-cell title="黑马程序员..." icon="search"></van-cell>
<van-cell title="黑马程序员..." icon="search"></van-cell>
<van-cell title="黑马程序员..." icon="search"></van-cell>
<van-cell title="黑马程序员..." icon="search"></van-cell>
<van-cell title="黑马程序员..." icon="search"></van-cell>
</div>
</template>
<script>
export default {
name: 'SearchSuggestion',
components: {},
props: {},
data () {
return {}
},
computed: {},
watch: {},
created () {},
mounted () {},
methods: {}
}
</script>
<style scoped lang="less"></style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
3、创建 src/views/search/components/search-result.vue
<template>
<div class="search-result">
<van-list
v-model="loading"
:finished="finished"
finished-text="没有更多了"
@load="onLoad"
>
<van-cell v-for="item in list" :key="item" :title="item" />
</van-list>
</div>
</template>
<script>
export default {
name: 'SearchResult',
components: {},
props: {},
data () {
return {
list: [],
loading: false,
finished: false
}
},
computed: {},
watch: {},
created () {},
mounted () {},
methods: {
onLoad () {
// 异步更新数据
// setTimeout 仅做示例,真实场景中一般为 ajax 请求
setTimeout(() => {
for (let i = 0; i < 10; i++) {
this.list.push(this.list.length + 1)
}
// 加载状态结束
this.loading = false
// 数据全部加载完成
if (this.list.length >= 40) {
this.finished = true
}
}, 1000)
}
}
}
</script>
<style scoped lang="less"></style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
4、在search/index.vue
中导入,注册,使用
import SearchResult from './components/search-result'
import SearchHistory from './components/search-history'
import SearchSuggestion from './components/search-suggestion'
2
3
components: {
SearchResult,
SearchHistory,
SearchSuggestion
}
2
3
4
5
<!-- 搜索结果 -->
<search-result />
<!-- /搜索结果 -->
<!-- 联想建议 -->
<search-suggestion />
<!-- /联想建议 -->
<!-- 搜索历史记录 -->
<search-history />
<!-- /搜索历史记录 -->
2
3
4
5
6
7
8
9
10
11
# 3.4、条件显示
1、在 data
中添加数据用来控制搜索结果的显示状态
data () {
...
isResultShow: false
}
2
3
4
2、在模板中绑定条件渲染
<!-- 搜索结果 -->
<search-result v-if="isResultShow" />
<!-- /搜索结果 -->
<!-- 联想建议 -->
<search-suggestion v-else-if="searchText" />
<!-- /联想建议 -->
<!-- 搜索历史记录 -->
<search-history v-else />
<!-- /搜索历史记录 -->
2
3
4
5
6
7
8
9
10
11
完成的页面模板
<template>
<div class="search-container">
<!-- 搜索栏 -->
<!--
Tips: 在 van-search 外层增加 form 标签,且 action 不为空,即可在 iOS 输入法中显示搜索按钮
-->
<form action="/">
<van-search
v-model="searchText"
show-action
placeholder="请输入搜索关键词"
background="#3296fa"
@search="onSearch"
@cancel="onCancel"
@focus="isResultShow=false"
/>
</form>
<!-- /搜索栏 -->
<!-- 搜索结果 -->
<search-result v-if="isResultShow" />
<!-- /搜索结果 -->
<!-- 联想建议 -->
<search-suggestion v-else-if="searchText" />
<!-- /联想建议 -->
<!-- 搜索历史记录 -->
<search-history v-else />
<!-- /搜索历史记录 -->
</div>
</template>
<script>
import SearchResult from './components/search-result'
import SearchHistory from './components/search-history'
import SearchSuggestion from './components/search-suggestion'
export default {
components: {
SearchResult,
SearchHistory,
SearchSuggestion
},
props: {},
data () {
return {
searchText:'', // 输入框绑定的数据
isResultShow:false // 搜索结果是否展示
}
},
computed: {},
watch: {},
created () {},
mounted () {},
methods: {
// 确定事件 【键盘按下了回车键或者手机键盘按下了搜索键/完成键】
onSearch(val){
console.log("确定了");
console.log(val)
// 显示搜索结果
this.isResultShow = true
},
// 取消事件 【点击了取消按钮或者关闭图标】
onCancel(){
this.$router.back();
}
}
}
</script>
<style scoped lang="less">
.search-container {
padding-top: 108px;
.van-search__action {
color: #fff;
}
.search-form {
position: fixed;
top: 0;
left: 0;
right: 0;
z-index: 1;
}
}
</style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
# 4、实现功能
# 4.1、搜索联想建议
基本思路:
- 当搜索框输入内容的时候,请求加载联想建议的数据
- 将请求得到的结果绑定到模板中
# 4.1.1 基本功能
一、将父组件中搜索框输入的内容传给联想建议子组件
二、在子组件中监视搜索框输入内容的变化,如果变化则请求获取联想建议数据
三、将获取到的联想建议数据展示到列表中
1、获取并监视内容变化
父组件
search/index.vue
里面通过自定义属性search-text
注入输入框内容<!-- 搜索建议 --> <search-suggestion v-else-if="searchText"="isResultShow" :search-text="searchText" /> <!-- /搜索建议 -->
1
2
3子组件
search-suggestion.vue
里面定义props
接收数据props: { // 接收输入框数据 searchText: { type: String, required: true } }
1
2
3
4
5
6
7使用
watch
去监听searchText
的数据变化watch:{ searchText:{ // 当 searchText 发生改变的时候就会调用 handler 函数 // 注意:handler 函数名称是固定的 handler(value){ console.log(value) }, immediate:true // 该回调将会在侦听开始之后被立即调用 } }
1
2
3
4
5
6
7
8
9
10
2、请求获取展示数据
定义请求方法
api/search.js
/** * 用户相关请求模块 */ import request from '@/utils/request' export const getSearchSuggestions = q => { return request({ method: 'GET', url: '/v1_0/suggestion', params: { q } }) }
1
2
3
4
5
6
7
8
9
10
11
12
13
14search-suggestion.vue
中导入使用import { getSearchSuggestions } from '@/api/search'
1data
定义存储数据变量data () { return { suggestions: [], // 联想建议数据列表 } }
1
2
3
4
5methods
定义获取数据方法methods: { // 定义获取数据方法 async loadSearchSuggestions (q) { try { const { data } = await getSearchSuggestions(q) this.suggestions = data.data.options } catch (err) { this.$toast('数据获取失败,请稍后重试') } } }
1
2
3
4
5
6
7
8
9
10
11watch
中去监听调用获取数据watch:{ searchText:{ // 当 searchText 发生改变的时候就会调用 handler 函数 // 注意:handler 函数名称是固定的 handler(value){ this.loadSearchSuggestions(value) }, immediate:true // 该回调将会在侦听开始之后被立即调用 } }
1
2
3
4
5
6
7
8
9
10渲染展示
<van-cell icon="search" v-for="(text, index) in suggestions" :key="index" :title="text" > </van-cell>
1
2
3
4
5
6
7
# 4.1.2 防抖优化
1、安装 lodash
- 工具函数lodash文档地址:https://lodash.com/docs/4.17.15
- 防抖函数:https://lodash.com/docs/4.17.15#debounce
# yarn add lodash
npm i lodash
2
2、防抖处理
// lodash 支持按需加载,有利于打包结果优化
import { debounce } from "lodash"
2
不建议下面这样使用,因为这样会加载整个模块。
import _ from 'lodash' _.debounce()
1
2
watch: {
searchText: {
// debounce 函数
// 参数1:一个函数
// 参数2:延迟时间,单位是毫秒
// 返回值:防抖之后的函数
// debounce(函数,时长) 返回一个防抖函数
handler: debounce(function (value) {
this.loadSearchSuggestions(value)
}, 200),
immediate: true // 该回调将会在侦听开始之后被立即调用
}
},
2
3
4
5
6
7
8
9
10
11
12
13
# 4.1.3 搜索关键字高亮
1、思路分析
如何将字符串中的指定字符在网页中高亮展示?
"Hello World";
将需要高亮的字符包裹 HTML 标签,为其单独设置颜色。
"Hello <span style="color: red">World</span>"
在 Vue 中如何渲染带有 HTML 标签的字符串?
data () {
return {
htmlStr: 'Hello <span style="color: red">World</span>'
}
}
2
3
4
5
<div>{{ htmlStr }}</div>
<div v-html="htmlStr"></div>
2
如何把字符串中指定字符统一替换为高亮(包裹了 HTML)的字符?
const str = "Hello World"
// 结果:<span style="color: red">Hello</span> World
"Hello World".replace('Hello', '<span style="color: red">Hello</span>')
// 需要注意的是,replace 方法的字符串匹配只能替换第1个满足的字符
// <span style="color: red">Hello</span> World Hello abc
"Hello World Hello abc".replace('Hello', '<span style="color: red">Hello</span>')
// 如果想要全文替换,使用正则表达式
// g 全局
// i 忽略大小写
// <span style="color: red">Hello</span> World <span style="color: red">Hello</span> abc
"Hello World Hello abc".replace(/Hello/gi, '<span style="color: red">Hello</span>')
2
3
4
5
6
7
8
9
10
11
12
13
14
2、实现过程
1、在 methods 中添加一个方法处理高亮
highlight (text) {
const highlightStr = `<span style="color:red;">${this.searchText}</span>`
// 正则表达式 // 中间的内容都会当作匹配字符来使用,而不是数据变量
// 如果需要根据数据变量动态的创建正则表达式,则手动 new RegExp
// RegExp 正则表达式构造函数
// 参数1:匹配模式字符串,它会根据这个字符串创建正则对象
// 参数2:匹配模式,要写到字符串中
const reg = new RegExp(this.searchText, 'gi')
return text.replace(reg, highlightStr)
}
2
3
4
5
6
7
8
9
10
11
2、然后在search-suggestion.vue
中绑定调用 v-html="highlight(item)"
<!-- 联想建议 -->
<van-cell-group v-else-if="searchContent">
<van-cell v-for="(item,index) in suggestions" :key="index" icon="search">
<div slot="title" v-html="highlight(item)"></div>
</van-cell>
</van-cell-group>
<!-- /联想建议 -->
2
3
4
5
6
7
注意: 这里有同学可能有疑问,为什么不使用过滤器,因为我们这里必须使用v-html
解析html
字符串,而过滤器和指令没有办法一起使用; 其次有同学可能还有疑问,为什么不在赋值数据前就把数据处理好,这是因为如果那么做就破坏了数据的原始内容,我们后期需要将原始内容传递给搜索结果。
# 4.2、搜索结果
基本思路:
找到数据接口
根据搜索关键字去请求获取数据
将数据展示到模板中
# 4.2.1 传递搜索内容
需求分析: 当我们点击某个搜索建议项的时候,我们应该把点击的内容传递给父组件,放入到输入框中去。
1、在search-suggestion.vue
中给van-cell
增加点击事件,触发自定事件search
<van-cell
icon="search"
v-for="(text, index) in suggestions"
:key="index"
@click="$emit('search', text)"
>
2
3
4
5
6
2、父组件search/index.vue
中的监听自定义事件,而监听的事件函数恰巧就可以直接用onSearch
<!-- 联想建议 -->
<search-suggestion
v-else-if="searchText"
:search-text="searchText"
@search="onSearch"/>
2
3
4
5
onSearch (val) {
// 更新文本框内容
this.searchText = val
// 打开搜索结果
this.isResultShow = true
}
2
3
4
5
6
**注:**很多同学好奇为什么这里也是用onSearch
,因为本身点击了某个搜索建议就和输入了某个内容点击只有的效果是一致的,最终都是要执行onSearch
所以我们直接关联了onSearch
事件函数。
3、在父组件search/index.vue
中将输入框的内容传递给搜索结果组件search-result.vue
<!-- 搜索结果 -->
<search-result
v-if="isResultShow"
:search-text="searchText"
/>
<!-- /搜索结果 -->
2
3
4
5
6
search-result.vue
中接收数据
props: {
// 接收传递进入的数据
searchText: {
type: String,
required: true
}
}
2
3
4
5
6
7
# 4.2.2 请求和渲染
1、在 api/serach.js
添加封装获取搜索结果的请求方法
/**
* 获取搜索结果
*/
export function getSearchResult(params) {
return request({
method: "GET",
url: "/v1_0/search",
params
})
}
2
3
4
5
6
7
8
9
10
2、search-result.vue
中导入方法,请求获取数据
<script>
// 导入请求方法
import { getSearchResult } from '@/api/search'
export default {
name: 'SearchResult',
components: {},
props: {
searchText: {
type: String,
required: true
}
},
data () {
return {
list: [], // 定义存储变量
loading: false,
finished: false,
page: 1,
perPage: 20,
error: false
}
},
computed: {},
watch: {},
created () {},
mounted () {},
methods: {
async onLoad () {
try {
// 1. 请求获取数据
const { data } = await getSearchResult({
page: this.page, // 页码
per_page: this.perPage, // 每页大小
q: this.searchText // 查询关键词
})
// 测试代码,可以删除
if (Math.random() > 0.5) {
JSON.parse('dsnajndjsa')
}
// 2. 将数据添加到数组列表中
const { results } = data.data
this.list.push(...results)
// 3. 将本次加载中的 loading 关闭
this.loading = false
// 4. 判断是否还有数据
if (results.length) {
// 如果有,则更新获取下一个数据的页码
this.page++
} else {
// 如果没有,则将加载状态 finished 设置为结束
this.finished = true
}
} catch (err) {
// 展示加载失败的提示状态
this.error = true
// 加载失败了 loading 也要关闭
this.loading = false
}
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
3、模板绑定
<van-cell
v-for="(article, index) in list"
:key="index"
:title="article.title"
/>
2
3
4
5
# 4.3、搜索历史记录
# 4.3.1 添加历史记录
当发生搜索的时候我们才需要记录历史记录。
1、在search/index.vue
中 data
添加一个数据用来存储历史记录
data () {
return {
...
searchHistories: []
}
}
2
3
4
5
6
2、在触发搜索的时候,记录历史记录
onSearch (val) {
// 更新文本框内容
this.searchText = val
// 存储搜索历史记录
// 要求:不要有重复历史记录、最新的排在最前面
const index = this.searchHistories.indexOf(val)
if (index !== -1) {
this.searchHistories.splice(index, 1)
}
this.searchHistories.unshift(val)
// 渲染搜索结果
this.isResultShow = true
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 4.3.2 展示历史记录
需要父子通信,将数据传递给search-history.vue
去渲染使用
1、父组件search/index.vue
传递数据
<!-- 搜索历史记录 -->
<search-history :search-histories="searchHistories" v-else />
<!-- /搜索历史记录 -->
2
3
2、子组件search-history.vue
接收数据
props:{
// 搜索历史,接收外部传入数据
searchHistories:{
type:Array,
required:true
}
}
2
3
4
5
6
7
3、渲染数据
<!-- 历史记录 -->
<van-cell-group v-else>
<van-cell title="历史记录">
<van-icon name="delete" />
<span>全部删除</span>
<span>完成</span>
</van-cell>
<van-cell
:title="item"
v-for="(item, index) in searchHistories"
:key="index"
>
<van-icon name="close"></van-icon>
</van-cell>
</van-cell-group>
<!-- /历史记录 -->
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 4.3.3 删除历史记录
基本思路:
- 给历史记录中的每一项注册点击事件
- 在处理函数中判断
- 如果是删除状态,则执行删除操作
- 如果是非删除状态,则执行搜索操作
1、处理删除相关元素的展示状态
- 在 data 中添加一个数据用来控制删除相关元素的显示状态
data () {
return {
...
isDeleteShow: false
}
}
2
3
4
5
6
- 绑定使用
<template>
<div class="search-history">
<van-cell title="搜索历史">
<div v-if="isDeleteShow">
<span>全部删除</span>
<span @click="isDeleteShow = false">完成</span>
</div>
<van-icon v-else name="delete" @click="isDeleteShow = true" />
</van-cell>
<van-cell
:title="item"
v-for="(item, index) in searchHistories"
:key="index"
>
<van-icon v-show="isDeleteShow" name="close" />
</van-cell>
</div>
</template>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2、处理删除操作
<!--全部删除按钮绑定事件,触发自定义事件-->
<span @click="$emit('clear-search-histories')">全部删除</span>
<!--每个van-cell点击的时候删除-->
<van-cell
:title="item"
v-for="(item, index) in searchHistories"
:key="index"
@click="onSearchItemClick(item, index)"
>
2
3
4
5
6
7
8
9
10
onHistoryClick (item, index) {
// 如果是删除状态,则执行删除操作
if (this.isDeleteShow) {
// 删除历史记录
this.searchHistories.splice(index, 1)
} else {
// 执行搜索操作
this.$emit('search',item)
}
}
2
3
4
5
6
7
8
9
10
父组件search/index.vue
监听自定义事件,执行搜索操作和删除全部操作
<!-- 搜索历史记录 -->
<search-history
v-else
:search-histories="searchHistories"
@clear-search-histories="searchHistories = []"
@search="onSearch"
/>
<!-- /搜索历史记录 -->
2
3
4
5
6
7
8
# 4.3.4 数据持久化
1、在search/index.vue
中利用 watch
监视统一存储数据
// 导入本地存储封装方法
import { setItem, getItem } from '@/utils/storage'
2
watch: {
searchHistories (val) {
// 同步到本地存储
setItem('serach-histories', val)
}
}
2
3
4
5
6
2、初始化的时候从本地存储获取数据
data () {
return {
...
searchHistories: getItem('serach-histories') || [],
}
}
2
3
4
5
6